In Python, repr() is a built-in function that returns a string representation of an object. This representation is meant for developers to understand and debug code. It provides a detailed, unambiguous string that can often be used to recreate the object. While str() is used for creating readable output for users, repr() is designed to give more detailed information useful for debugging.
What Is repr in Python? (With Simple Example)
The repr() function in Python returns a string that represents an object in a way that it can be understood by developers. This string often includes extra details such as quotes for strings or additional escape characters.
Here’s a simple example:
python
CopyEdit
text = “Hello, world!”
print(repr(text)) # Output: ‘Hello, world!’
In this example, the repr() function returns ‘Hello, world!’ with single quotes, making it clear that the output is a string. This helps developers see the exact representation of an object, including special characters if present.
Why Do We Use repr() in Python?
The repr() function is used primarily for debugging and logging. It provides a clear, precise representation of an object so that developers can understand its structure. Here are some key reasons why repr() is useful:
- It helps debug complex objects.
- It provides a detailed string representation of an object.
- It allows recreating objects by evaluating the returned string using eval().
- It makes logging and error messages more informative.
For example, if an object contains special characters, repr() ensures they are displayed correctly:
python
CopyEdit
text = “Hello\nWorld”
print(str(text)) # Output: Hello
# World
print(repr(text)) # Output: ‘Hello\nWorld’
In this case, repr() clearly shows the \n newline character, while str() directly prints the multi-line text.
How Is repr() Different from str()?
Python has two functions for converting objects to strings: repr() and str(). Though they may seem similar, they serve different purposes.

repr() Gives More Details
The repr() function is designed for developers. It provides a string what is repr in python that includes details useful for debugging.
Example:
python
CopyEdit
print(repr(3.14)) # Output: ‘3.14’
print(repr(“Python”)) # Output: “‘Python'”
Here, repr() adds extra quotes around the string, making it clear that it is a string.
str() Is for Humans, repr() Is for Debugging
The str() function is meant for human-readable output, while repr() is designed to provide more detailed technical information.
Example:
python
CopyEdit
import datetime
now = datetime.datetime.now()
print(str(now)) # Output: ‘2025-02-24 15:30:45.123456’ (human-readable)
print(repr(now)) # Output: ‘datetime.datetime(2025, 2, 24, 15, 30, 45, 123456)’ (detailed)
repr() Often Includes Quotes
For string objects, repr() often includes quotes, while str() does not.
python
CopyEdit
word = “Python”
print(str(word)) # Output: Python
print(repr(word)) # Output: ‘Python’
This small difference helps when debugging complex strings, ensuring clarity.
How to Use repr() in Python? (Code Examples)
Using repr() in Python is simple. You can pass any object to it, and it will return a detailed string representation.
Here are some common use cases:
Using repr() with Different Data Types
python
CopyEdit
num = 100
text = “Hello”
lst = [1, 2, 3]
print(repr(num)) # Output: ‘100’
print(repr(text)) # Output: “‘Hello'”
print(repr(lst)) # Output: ‘[1, 2, 3]’
Using repr() in Logging
python
CopyEdit
import logging
logging.basicConfig(level=logging.DEBUG)
text = “Debugging string\nwith new line”
logging.debug(repr(text))
Here, repr() ensures that the \n (newline) character is visible in logs.
Does Every Object Have repr() in Python?
Yes! Every object in Python has a repr() method. If a custom object does not have a defined __repr__ method, Python provides a default one, which includes the memory address of the object.
Example:
python
CopyEdit
class Example:
pass
obj = Example()
print(repr(obj))
# Output: ‘<__main__.Example object at 0x7f12345678>’
Since we haven’t defined a __repr__ method in the class, Python provides a default representation showing the object’s type and memory location.
Creating a Custom repr() in a Python Class
You can customize the output of repr() for your own classes by defining the __repr__ method inside a class.
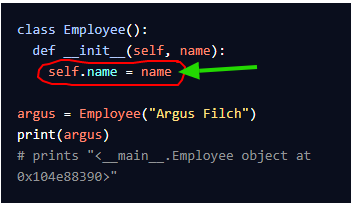
Define a Class
python
CopyEdit
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
Return a Useful String
To make debugging easier, we define __repr__ to return useful details about the object.
python
CopyEdit
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __repr__(self):
return f”Person(name='{self.name}’, age={self.age})”
p = Person(“Alice”, 25)
print(repr(p))
# Output: “Person(name=’Alice’, age=25)”
Now, when we use repr(), we get a meaningful output that helps in debugging.
Common Mistakes When Using repr() (And How to Fix Them)
Even though repr() is useful, there are some common mistakes to avoid:
- Forgetting to Define __repr__ in Custom Classes
- If you don’t define __repr__, Python provides a generic one that may not be useful.
- Confusing repr() with str()
- Remember, repr() is for debugging, while str() is for user-friendly output.
- Using repr() on Large Data Objects Without Truncation
- Large objects can result in long outputs. Consider truncating output if necessary.
Example of truncation:
python
CopyEdit
data = list(range(1000))
print(repr(data[:10]) + ‘ …’) # Output: ‘[0, 1, 2, 3, 4, 5, 6, 7, 8, 9] …’
The Bottom Line
The repr() function in Python is a powerful tool for debugging and understanding objects. Unlike str(), which provides user-friendly output, repr() offers an unambiguous representation useful for developers. By defining a custom __repr__ method in classes, you can make debugging easier and improve code readability.